Embedding QuickSight Dashboards
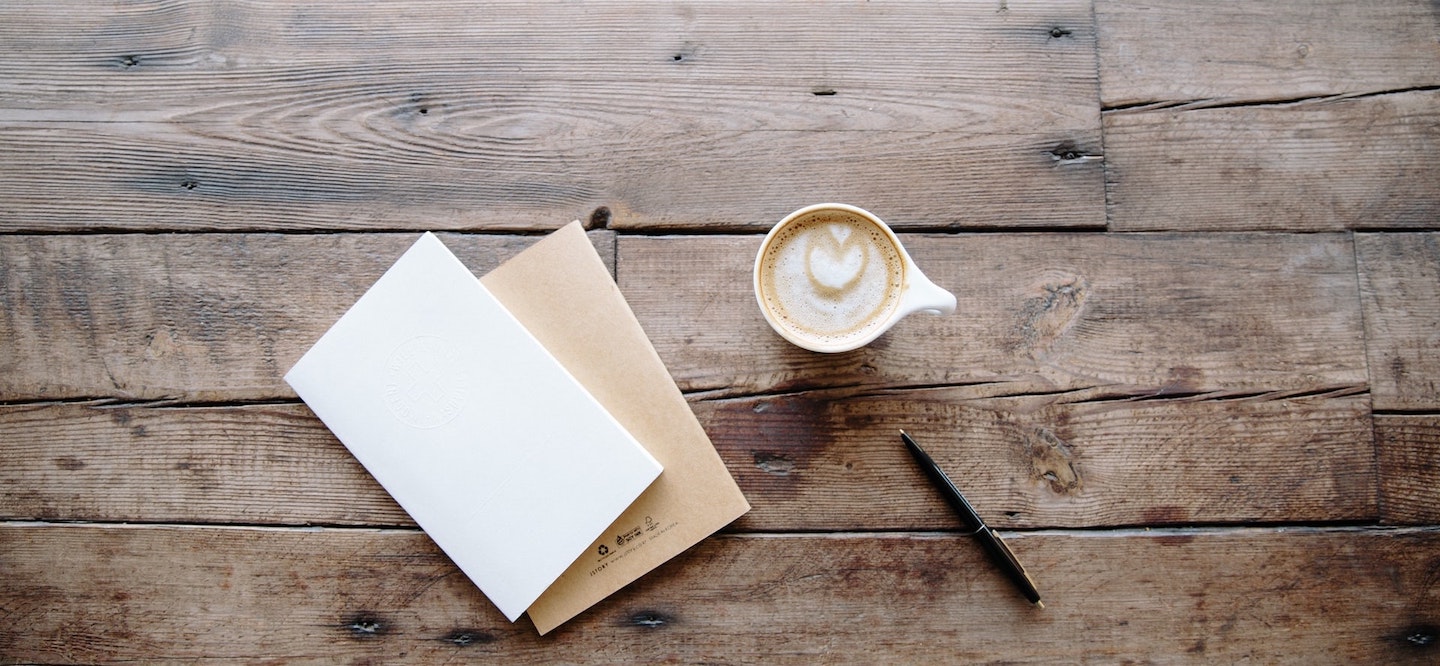
This article demonstrates how to embed Amazon QuickSight dashboards into your web application using API Gateway, AWS Lambda, Amazon Cognito, and Secrets Manager for secure integration.
Overview
Amazon QuickSight is a powerful business intelligence service that allows you to create interactive dashboards. Embedding QuickSight dashboards into your applications enables users to seamlessly interact with data visualizations without leaving the application.
This architecture demonstrates how to enable secure access to QuickSight dashboards using the following AWS services:
- API Gateway: Acts as an entry point for client requests.
- Cognito: Provides user authentication and token-based security.
- AWS Lambda: Manages custom logic for user migration and generating QuickSight embedded dashboard URLs.
- Secrets Manager: Securely stores database credentials for integration with RDS.
- RDS: Serves as the source database for QuickSight.
Here's the high-level architecture:
Architecture Breakdown
Key Components
Cognito:
- Users log in via Amazon Cognito User Pools.
- Cognito issues JWT tokens to authenticated users.
- Tokens are validated by the API Gateway before allowing access to the backend services.
API Gateway:
- Serves as the front door to the backend services.
- Handles requests for user migration and QuickSight embedded dashboard URLs.
- Verifies tokens using a Cognito Authorizer.
Lambda Functions:
-
UserMigration:
- Migrates users to the application and retrieves credentials from Secrets Manager to interact with the database.
-
QuickSightEmbedded:
- Generates signed URLs for embedding QuickSight dashboards.
Secrets Manager:
- Stores and retrieves RDS credentials securely.
RDS:
- Provides the underlying data source for QuickSight dashboards.
QuickSight:
- Hosts dashboards and provides the ability to embed them securely.
Implementation Steps
Step 1: Set Up Cognito User Pool
- Create a Cognito User Pool for user authentication.
- Enable App Clients to integrate with your web application.
- Configure custom attributes (if needed) to manage user-specific settings.
Step 2: Configure API Gateway
- Create an HTTP API in API Gateway.
- Set up a Cognito JWT Authorizer to validate tokens.
- Define routes for:
-
/login
→ Invokes the UserMigration Lambda function. -
/embedded-url
→ Invokes the QuickSightEmbedded Lambda function.
-
Step 3: Build Lambda Functions
UserMigration Function (/login
)
The UserMigration function:
- Authenticates users by validating credentials.
- Retrieves RDS credentials from Secrets Manager.
- Checks or updates the user database.
Here’s an example implementation:
import boto3
import json
import pymysql
secrets_client = boto3.client('secretsmanager')
def lambda_handler(event, context):
# Retrieve credentials from Secrets Manager
secret = secrets_client.get_secret_value(SecretId="rds/credentials")
credentials = json.loads(secret['SecretString'])
connection = pymysql.connect(
host=credentials['host'],
user=credentials['username'],
password=credentials['password'],
database=credentials['database']
)
# Process user migration logic here
body = json.loads(event['body'])
username = body['username']
password = body['password']
# Example SQL to check users
with connection.cursor() as cursor:
cursor.execute("SELECT * FROM users WHERE username = %s", (username,))
user = cursor.fetchone()
if user:
return {"statusCode": 200, "body": json.dumps({"message": "User exists"})}
else:
return {"statusCode": 401, "body": json.dumps({"message": "User not found"})}
QuickSightEmbedded Function (/embedded-url) The QuickSightEmbedded function:
Generates a signed QuickSight URL for embedding a dashboard. Accepts user-specific parameters to filter dashboard content.
import boto3
import json
quicksight_client = boto3.client('quicksight')
def lambda_handler(event, context):
# Extract user ID and dashboard details from the request
body = json.loads(event['body'])
user_arn = body['user_arn']
dashboard_id = body['dashboard_id']
# Generate QuickSight Embed URL
response = quicksight_client.get_dashboard_embed_url(
AwsAccountId="YOUR_ACCOUNT_ID",
DashboardId=dashboard_id,
IdentityType="QUICKSIGHT",
UserArn=user_arn,
SessionLifetimeInMinutes=60
)
return {
"statusCode": 200,
"body": json.dumps({"embed_url": response['EmbedUrl']})
}
Step 4: Integrate QuickSight with RDS
- Connect Amazon QuickSight to your RDS instance.
- Create datasets and dashboards using the connected data source.
- Ensure that IAM roles and permissions for Lambda and QuickSight are correctly configured.
Adding Security Layers
- Use AWS Certificate Manager to enable HTTPS on your API Gateway.
- Store sensitive credentials in Secrets Manager and avoid hardcoding them in your Lambda functions.
- Use IAM roles with least-privilege access to interact with QuickSight and RDS.
Conclusion
With this architecture, you can securely embed Amazon QuickSight dashboards into your web application. The combination of API Gateway, Lambda, and Cognito ensures that only authenticated users can access the dashboards while maintaining flexibility and scalability.
For detailed documentation, refer to Amazon QuickSight Embedding Guide.